Using adaptive bitrate streaming for video optimization
As online video consumption continues to rise, delivering high-quality video experiences to viewers is becoming increasingly important. One of the key challenges in video delivery is ensuring that the video streams smoothly and without interruptions, even when internet connection speeds fluctuate. This is where Adaptive Bitrate Streaming (ABS) comes in. By dynamically adjusting the quality of the video based on the viewer's internet connection speed, ABS ensures that the video streams seamlessly and without buffering.
To provide the best video experience we need to be responsive, adapting our content to our user's conditions, preserving the visual quality. Keeping the bitrate at the minimum and as possible, and always below the connection limit, is mandatory. In this regard, Adaptive Bitrate protocols provide an enhanced viewing experience, improving search ranking (SEO), and increasing viewers engagement.
Adaptive bitrate protocol
Embedding progressive video in HTML5 is a simple process. Similar to images, we just need to include a link to the video in progressive format, and it should work on any browser that supports the coding standard used. However, this approach has significant limitations since the video's quality is fixed regardless of the network connection quality. In situations with slow or congested networks, high-resolution videos may have poor quality or result in slow page speeds, video stalling, and re-buffering issues.
To overcome the limitations of progressive video in various connection speeds, we need to use adaptive bitrate (ABR) streaming protocols. These protocols split and encode videos into multiple files with different bitrates and adapt the quality to the available bandwidth. Popular ABR options include MPEG-DASH, HLS, and others.
HLS, an ABR protocol developed by Apple, uses multiple renditions of the video, each with a different bitrate, to adapt the quality to the connection's speed. Videos are encoded at different bitrates and resolutions in a bitrate ladder, and each rendition is split into multiple chunks. The protocol adjusts the requested bitrate automatically according to the available bandwidth, allowing it to avoid video re-buffering and stalling, common issues with slower connections.
HLS has extensive support, with native support in iOS and Android (when videos are encoded using H264) and support in Safari browsers for macOS. For other browsers and systems, a javascript library like hls.js is needed to negotiate bitrates.
Video per-title encoding
When encoding videos for HLS streaming, it's important to define a bitrate ladder (bitrates and resolutions) and chunk length. The resulting output includes a set of playlists, typically with a .m3u8 extension, and chunks, typically with a .ts extension. These playlists include a master playlist, additional playlists for each rendition, and all the chunks for each rendition. The master playlist specifies the bitrate ladder and the path to each rendition.
While Apple recommends a bitrate ladder with fixed bitrates and a 10-second length for chunks, this approach is far from optimal. It can result in poor quality for high dynamic videos and a waste of bandwidth for low dynamic videos. There is no one-size-fits-all solution for adjusting video bitrates, as it can lead to overweight for some videos and quality issues for others. In fact, the recommendation is directly useless for short videos, such as those used in ecommerce and marketing.
In general, the best approach is to apply per-title encoding and optimize the bitrate ladder by tuning the bitrates based on the content of each video. Video encoding parameters should be selected for each video content, and the results should be tested and tweaked accordingly to meet the specific requirements. By performing per-title encoding and analyzing each video to adapt the bitrate ladder, it's possible to balance compression and content for the best visualization experience across any device.
While HLS can increase the complexity of the transcoding pipeline and the front-end, it brings breakthrough improvements in the quality of experience. For high-resolution videos over 5 seconds long, we have seen clear benefits associated with HLS. When combined with fast H264 encoding, it also helps to limit the cost and latency of transcoding. Therefore, HLS with per-title encoding is definitely our format of choice for long videos.
Embedding HLS video in HTML
Typically, delivering HLS requires the use of a JavaScript library that implements the protocol, such as hls.js, to provide support for browsers that use the Media Source Extensions API. This enables us to utilize the default HTML5 video player while still ensuring cross-browser HLS support. By adding the following code, we can automatically check network speed and negotiate quality with the server, based on the playlist that details available renditions depending on resolution and bandwidth.
<video id="player"></video>
<script src="https://cdnjs.cloudflare.com/ajax/libs/hls.js/0.12.4/hls.min.js"></script>
<script>
var video = document.getElementById('player');
if (Hls.isSupported()) {
var hls = new Hls({ autoStartLoad: false });
hls.attachMedia(video);
hls.on(Hls.Events.MEDIA_ATTACHED, function () {
hls.loadSource('https://store.abraia.me/05bf471cbb3f9fa9ed785718e6f60e28/HLS-video/HLS_video-at-work/playlist.m3u8');
hls.on(Hls.Events.MANIFEST_PARSED, function (event, data) {
video.play();
});
});
} else {
// fallback
}
</script>
A more flexible approach involves separating the video visualization from the HTML code of the embedding page. This can be achieved by creating an HTML resource that contains the video player code and the video URL, which can then be embedded using an iframe. By doing so, we can easily customize the video experience for users, as well as optimize for SEO and analytics.
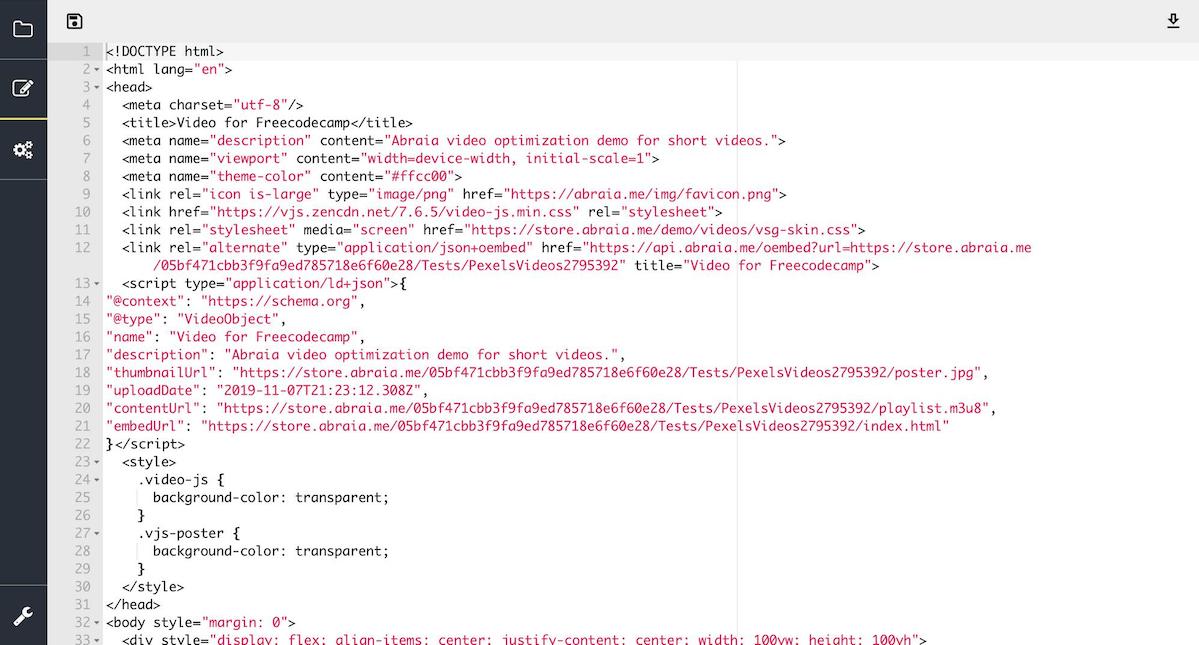
For instance, we can easily customize the video experience with videojs. It can play both progressive videos and HLS streams, and allows easy control over the visual appearance of the player using skins and a flexible configuration, enabling the use of autoplay or different video controls. The implementation code for the player may be as simple as shown in the following example:
<video id="player" class="video-js vjs-default-skin vjs-big-play-centered" width="100%" controls autoplay muted loop preload>
</video>
</div>
<script src="https://vjs.zencdn.net/7.6.5/video.min.js"></script>
<script>
const player = videojs('player', {
responsive: true,
fluid: true
});
player.src({
src: 'https://store.abraia.me/05bf471cbb3f9fa9ed785718e6f60e28/HLS-video/HLS_video-at-work/playlist.m3u8',
type: 'application/x-mpegURL'
});
</script>
Additionally, videojs also supports plugins, which enables tracking of video-related events (like play or pause actions) so we can include them in our own analytics. For instance, we may include the following code in our player using the corresponding plugin.
player.analytics({
defaultVideoCategory: 'Video',
events: [{
name: 'play',
label: 'Video-Title',
action: 'play',
}, {
name: 'pause',
label: 'Video-Title',
action: 'pause',
}, {
name: 'ended',
label: 'Video-Title',
action: 'ended',
}, {
name: 'error',
label: 'Video-Title',
action: 'error',
}]
});
And we will find the data collected on the tracked events available in our Google Analytics.
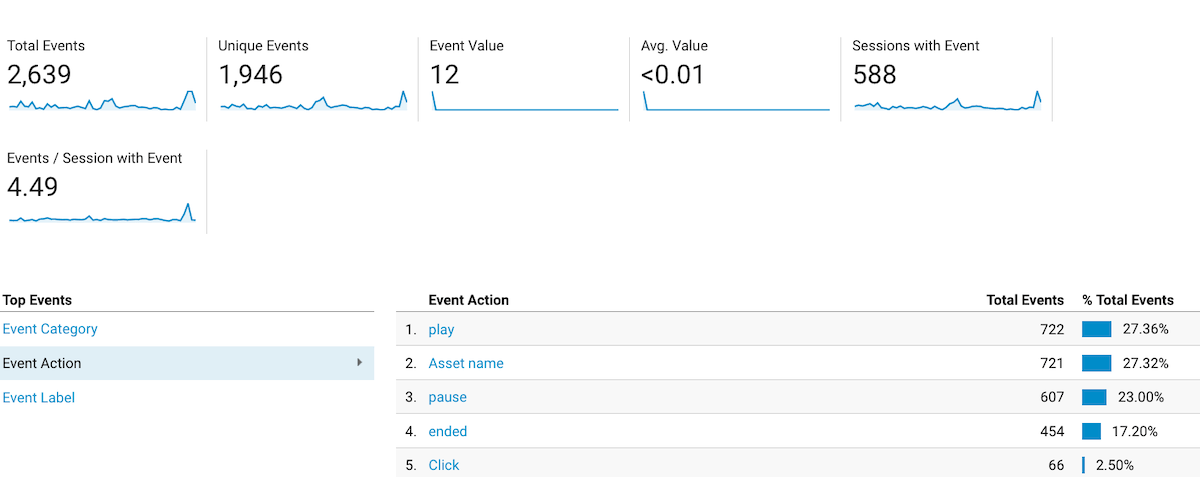
Then we insert this HTML document in the HTML code of the page where we want to embed the video using the HTML5 element iFrame with the source attribute pointing to the url the HTML to embed.
<div style="position:relative;width:100%;height:0;padding-bottom:56.25%;">
<iframe width="1920" height="1080" allowfullscreen
style="border:0;top:0;left:0;width:100%;height:100%;position:absolute;"
src="https://store.abraia.me/05bf471cbb3f9fa9ed785718e6f60e28/videoExample/index.html"></iframe>
</div>
With Abraia you can easily convert your videos to HLS ready to integrate into your website. You just need to put the generated assets on a cloud storage and deliver the content using your current CDN provider. We provide all the code to personalize and customize the aspect of the video player and the SEO and analytics code. You will take control of any aspect of your media experience and SEO in your website.